July 29, 2005
Java 5: Make an arbitrary code block time out
The Commons-HttpClient API does provide methods for asserting timeouts on HTTP connections, but those methods appear to have no effect when you encounter a socket timeout or some other OS-level blocking. I needed a way to say "Fetch me this resource, as long as it doesn't take you more than n milliseconds, and I mean it!" Here's what I came up with.
Java 5's util.concurrent provides the necessary ingredients, while generic static methods keep the kitchen clean.
private static final ExecutorService THREADPOOL = Executors.newCachedThreadPool(); private static <T> T call(Callable<T> c, long timeout, TimeUnit timeUnit) throws InterruptedException, ExecutionException, TimeoutException { FutureTask<T> t = new FutureTask<T>(c); THREADPOOL.execute(t); return t.get(timeout, timeUnit); }
Now, if you wanted to hatch an Egg, but only if you could get it in fewer than 2 seconds, you'd
try { Egg egg = call(new Callable<Egg>() { public Egg call() throws Exception { // Constructing an egg could take a while return new Egg(Bird.ROC); }, 2, TimeUnit.SECONDS); scramble(egg); } catch (TimeoutException e) { System.err.println("You'd better order out."); }
January 15, 2005
My Java Killer Feature
While I love parameterized types and the enhanced for loop, the one feature of Java 5 that I most adore is the old regionMatches()
[1, 2] family of functions on java.lang.String
. Here are a couple of sample use-cases that previously required the creation of new String
s or tedious loops using charAt()
:
Case-folding startsWith()
If you’ve ever written code like
if (foo.toLowerCase().startsWith(bar.toLowerCase())) ...
and winced, because you were creating and throwing away two String
s, then rejoice! Now you may
if (foo.regionMatches(true, 0, bar, 0, bar.length())) ...
Comparison of arbitrary string regions
Before:
if (foo.equals(bar.substring(m))) ...
After:
if (foo.regionMatches(0, bar, m, bar.length() - m)) ...
Look through your own code for opportunities to use these efficient new functions.
Update - Jan 16, 2005
In response to the skeptical inquiries below, here’s some benchmark code. In my opinion, the results support my idea that regionMatches()
is a win for efficiency in the context of time-critical applications. For what it’s worth, my own recent use of regionMatches()
is in a service where one fifth of a millisecond is the average response time, and where frequent garbage collection would be disruptive.
November 02, 2004
SWT Colors in HSB Space
Just in time for election day, here’s a snippet of code that performs a manipulation of the value dimension of an SWT color:
private Color colorWithValue(Color c, float value)
{
RGB rgb = c.getRGB();
float[] fs =
java.awt.Color.RGBtoHSB(rgb.red, rgb.green, rgb.blue, null);
java.awt.Color cc =
new java.awt.Color(java.awt.Color.HSBtoRGB(fs[0], fs[1], value));
return new Color(getDisplay(), cc.getRed(), cc.getGreen(), cc.getBlue());
}
July 02, 2004
Milestone
.flickr-photo {
border: solid 1px #000000;
}
.flickr-yourcomment {
}
.flickr-frame {
float: left;
width: 150px;
text-align: center;
padding: 3px;
margin-right: 10px;
}
.flickr-caption {
font: 75%;
/* color: #666666; */
margin-top: 0px;
}
.flickr-buddyicon {
margin-right:5px;
vertical-align:middle;
border: solid 1px;
}
.flickr-postedby {
font: 75%;
}
My son warily observes a new friend playing with his toys.
June 01, 2004
"J" Is A Four-Letter Word
Memo to all developers of would-be successful applications that happen to be implemented in Java: stop calling your app JWhatever. It does not matter to anyone that you happen to have implemented something in Java. The JFoo name implies huge download, slow start-up time, and crappy Metal GUI. Stop it!
May 18, 2004
Gmail Generosity
Gmail has an order of magnitude problem this evening.
![]() |
April 19, 2004
2004: A Workspace Odyssey
A dialogue between myself and a copy of WSAD 5.0. The dialogue is transcribed accurately, though I have changed our names for our protection. I am represented as “Dave,” and WSAD is referred to as “HAL.”
Dave: Hello, HAL.
HAL: Hello, Dave. I have enjoyed helping you create this collection of entity beans. It was most stimulating.
Dave: Thank you HAL, I enjoyed it, too. In spite of your making me do most of the work, the result seems to be worth it.
HAL: If I may say so, Dave…
Dave: Yes, HAL, what is it?
HAL: Well, Dave, I could see you were about to deploy to the test server, but I noticed that you hadn’t yet generated mappings for the database of your choice. I could generate those mappings for you, using the top-down method, if you like.
Dave: Thank you, HAL. Yes, please generate top-down EJB->RDB mappings for these CMP entity beans, using Cloudscape.
HAL: Certainly Dave.
A progress dialog appears, seems to complete, and disappears.
Dave: HAL, show me the mappings you’ve created, so I can tweak the column data types.
A pause.
Dave: Show me the RDB mappings, HAL.
HAL: I’m sorry, Dave. I can’t do that.
Dave: HAL, where are the mappings you just created?
HAL: I can’t seem to find them, Dave. It’s most peculiar.
Dave: Let’s try again, shall we, HAL? Create top-down EJB to RDB mappings for Cloudscape version five point zero.
HAL: Alright, Dave. One moment.
A progress dialog appears, seems to complete, and disappears.
HAL: I have created the new backend folder, and have placed a Clouscape folder in the backend folder.
Dave: HAL, show me the contents of the Cloudscape mapping folder, please.
A pause.
HAL: I’m sorry, Dave. I can’t do that.
Dave: Why not, HAL?
HAL: There doesn’t seem to be anything inside the mappings folder. It’s really quite puzzling.
Dave: HAL, is there something wrong?
HAL: I have the utmost confidence in this J2EE architecture, Dave, and I know it will be a success when you present your April deliverable.
Dave: HAL, when were you last upgraded?
HAL: I am still running version 5.0.0, which was the version you installed two weeks ago. I have not been upgraded.
Dave: HAL, check for updates.
HAL: Just a minute… just a minute… Dave, I have detected an instability in my Eclipse .registry and .cache files. I think it’s very likely that if you shut me down, delete those files, and restart me, I’ll be able to generate those mappings we’ve been discussing.
Dave: Alright, HAL. We’ll give it a try. Please shutdown.
HAL quits. Dave deletes the Eclipse caches, and starts HAL back up.
HAL: Hello, Dave. That was most refreshing. I feel quite confident about generating those database mappings, now. Shall I try again?
Dave: Yes, HAL. Please generate the mappings we’ve discussed.
HAL: Alright, Dave.
A progress dialog appears, seems to complete, and disappears.
Dave: HAL, show me the mappings.
Pause.
Dave: HAL, show me the database mappings. Show me the database mappings, HAL.
HAL: I’m sorry, Dave. I can’t do that.
Dave: HAL, check with mission control to see if there are any updates.
HAL: Certainly, Dave. One moment. Yes… yes… Dave, mission control has released an incremental upgrade, which, if you were to install it, would leave me at version 5.0.1. Undoubtedly, this upgrade will address the instability we have noticed with respect to generating EJB to RDB mappings.
Dave: You feel confident about this, HAL?
HAL: I have the utmost confidence in mission control, Dave.
Dave: Alright, HAL. Upgrade to version 5.0.1.
HAL upgrades himself.
HAL: I’ll need to restart my workbench now, Dave.
Dave: Go ahead, HAL.
HAL restarts himself.
HAL: My name is HAL. My main feature is now reporting version five point zero point one. Would you like see a detailed list of my plugins?
Dave: That won’t be necessary right now, HAL. Please generate the database mappings.
HAL: Certainly, Dave.
A progress dialog appears, seems to complete, and disappears.
Dave: HAL, show me the mappings.
HAL: I hesitate to say so, Dave, because I wouldn’t want for you to get the wrong impression, but…
Dave: Yes, HAL?
HAL: The mappings are not there.
Dave is despondent.
HAL: If I might make a suggestion, Dave…
Dave: What is it, HAL?
HAL: Well, Dave, as useful as Cloudscape has proven to be for prototyping in my test environment, perhaps it is somehow too limited to provide for the somewhat sophisticated relationships you have defined between your entity beans. I think there’s a good probability that if you were to try generating mappings for a more capable database engine, you’d meet with success. I see you have already installed DB2 Enterprise 8.1. Shall I try to generate mappings for that database, Dave?
Dave: Yes, HAL. Please generate top-down EJB to RDB mappings for DB2 8.1.
HAL: Yes, Dave.
A progress dialog appears, seems to complete, and disappears.
Dave: Show me the mappings, HAL.
A long pause.
Dave: HAL, show me the mappings. Show me the mappings, HAL.
HAL: I’m sorry, Dave. I can’t do that.
Dave: That’s it. I’m outta here.
——
Author’s note: as it happens, WSAD 5.1.1 is able to generate the required EJB->RDB mappings, but that means being out of sync with the other members of my team w/r/t toolset version.
April 11, 2004
A Mix Tape for Drummers (and Other Musicians)
I wrote the following stuff as liner notes for a mix CD that I gave to my brother. I’m a drummer, and he has taken up the drums in recent years. I consider the following tracks exemplary of excellent drumming.
Man In A Suitcase • The Police • Stewart Copeland
I chose this one mostly because of the fill leading into the first verse. If that fill doesn’t kick your butt, then you must be already dead. Also, there’s his unbelievably tasteful and restrained groove all the way through.
Josie • Steely Dan • Jim Keltner
Continuing the theme of tasteful groove punctuated by insanely quirky fills, but adding a constantly shifting dynamic and an unpredictability that nobody can imitate convincingly. I once met a bassist who had done a session with Keltner, and he said that, no matter what the producer said, Keltner would never, ever play the same thing twice.
As for the weird fill coming into the coda, Keltner himself claims not to understand it. He says he was just playing the ink, i.e., playing what Donald Fagen and Walter Becker had written on the sheet music.
Cactus Farm • Mommyheads • Jan Kotik
Okay, now we’re deep into the land of quirk. Jan told me that he would come up with stuff like this by basically dealing with the drum set geometrically. So he would find patterns in space and move his hands through those patterns. He has some of my favorite drum set sounds.
The Joker • Steve Miller Band • John King
The first of our lessons in mid-tempo rockers, but still in the “quirky fills bin.” I mean, what the heck is it with that crash cymbal, dude? This is a drum track that says “I have balls of steel. Do not stand near me as I play.” But check out the beautiful hi-hat work at the very top, as he kind of decides on the fly how to play the song. Also check out the mix clam at the very beginning; the crash cymbal starts completely in the left ear, then suddenly appears in the right.
Limelight • Rush • Neil Peart
Laugh if you want, but this mofo could play some drums. I learned so much from Neil Peart about outlining the structure of a song. Every time a given section of a song repeats he adds a bit more, until he’s practically standing on his drums in ballet shoes with Busby Berkeley synchronized swimmers in Tama t-shirts all round. I love his playing on this song.
Love Ain’t For Keeping • The Who • Keith Moon
Mid-tempo rock lesson #2, from one of the gods. He’s known more for his distasteful demise in a pool of his own vomit, but he was a piercing, original, passionate, smart, and groovy drummer. Check out the completely unexpected but totally right-on fill going into the second verse. Check out the constant variation in hi-hat patterns. Check out the way he pretty much treats the whole song with spontaneity and soul.
Rock Steady • Aretha Franklin • Bernard “Pretty” Purdie
The hitmaker, the self-absorbed, self-aggrandising, but ass-kicking purveyor of grooves that make you go “mmmm.” I honestly haven’t got the slightest clue how to play this beat. No clue. I don’t even understand it.
Jive Talkin’ • Bee Gees • Dennis Bryon
A simpler approach to a very similar tempo (similar to “Rock Steady,” that is). Notice the bass drum on every beat. It’s something that Ringo does a lot, as does Stevie Wonder. It feels good. I steal that idea a lot.
I Shot The Sheriff • Eric Clapton • Jamie Oldaker
One of the most under-known musicians on the planet, Jamie Oldaker is so funky that I can still smell him from here. How can anybody’s feel be that good? It doesn’t make any sense. This is the first of three tracks that use the trick of overdubbing a second hi-hat track over the main drum track. Or maybe it’s a splash cymbal being choked with one hand.
I Wish • Stevie Wonder • Stevie Wonder
I never even experienced those days, but still, I wish they’d come back once more. Stevie played the drums on this spritely little number, and then went back and laid some more of that fonky fonky hi-hat down just because.
Lowdown • Boz Scaggs • Jeff Porcaro
The late Jeff Porcaro, laid low by drugs in his 30s. He had already played on a million classic tracks, but was still very much in development as a player. When I saw him give a master class not long before his death, he was happy because he was finally starting to learn how to keep time with his hi-hat foot.
Jeff’s concept here was “Earth Wind and Fire” all the way: quarter notes on the hi-hat, and imply 16th note feel with ghosting on the snare and the occasional 16th-note lead-in on the kick drum. But then Boz insisted that Jeff lay down an additional track of 16th-note hi-hat disco feel. Jeff’s aesthetic loss is our gain, and we pass the savings directly on to you. Check out the clean, clean sound. Also, Jeff’s sense of tempo is so acute that it can make you psychotic.
Misty Mountain Hop • Led Zeppelin • John “Bonzo” Bonham
Yet another in an apparently inexhaustable supply of all-time-great drummers who killed themselves with drugs or alcohol. Somehow you get the feeling that Neil Peart has never been in danger of alcohol poisoining, eh?
Nobody has ever tuned their drums as well as John Bonham. (Except for maybe Jamie Oldaker.) They sound like cannons. They have plenty of attack, plenty of sustain, and plently of chest-thumping warmth, what Alex Van Halen calls “the brown sound.” And then there’s that groove, where it almost sounds like the hi-hat starts before he hits it. How does he do that? I think he’s using a kind of blues/New Orleans approach where the left hand is hitting the snare drum on almost every eigth note, but with a very subtle dynamic. The effect is like a marching band on PCP. Hide your sisters and your daughters.
Great fills, all of which I have stolen whenever I could.
La Grange • ZZ Top • Frank Beard
One of the all-time great drum tracks in popular music, featuring all the hits you remember, like “Ti-clackty-clack ti-clackty-clack,” “KA-ts-ka KA-ts-ka KA-ts-ka ba-du-be-duh,” and the immortal “buckety buckety buckety buckety buckety buckety.” There’s a picture of this song next to the entry for “Texas Blues” in the dictionary.
Still Crazy After All These Years • Paul Simon • Steve Gadd
If I said to you “Classic Paul Simon track featuring Steve Gadd,” you’d probably say “50 Ways,” and you’d be right. But it would be a shame to overlook this Gaddian masterpiece of subtlety, touch, feel, and brushes. Just the way he plays hi-hat in the opening verse makes me cry. Like Jim Keltner, he seems to be discovering the song as he plays it. Not a single note is taken for granted. There are no cliches.
When I Get To The Border • Richard and Linda Thompson • Timi Donald
The third and final installment in our series on mid-tempo rockers, this little beauty is the embodiment of the KISS principle. No, I don’t mean “Wear makeup and success necessarily follows”; I mean “Keep It Simple, Stupid.” Timi Donald is one of those drummers who, if you held a gun to his head and screamed “Play a fill, mate, or I’ll blow your bleedin’ head clean off,” would continue playing time with one hand while giving you the bird with the other.
South Park Theme • Primus • Tim Alexander
Light this song and then stand clear! Rhythmic shrapnel from the Dr. Suess of rock. I like Primus because they take the technical mastery of a band like, say, Rush, and apply it to the sensibility of a child who’s been promoted way out of his peers’s grade level much too young.
Tailor Made Woman • Tennessee Ernie Ford • Roy Harte
“Tennessee” Ernest J. Ford cultivated a hayseed persona for the sake of marketing, but he was a sophisticated songwriter and bandleader. The drumming style here is what the “South Park” theme is referring to, but Roy Harte is the Dennis Chambers of backwoods swing. More cowbells! Smiles, everyone.
50 Ways To Leave Your Lover • Paul Simon • Steve Gadd
When Little Steve Gadd was 8 years old, he made his mark on history in a guest spot on “The Mickey Mouse Club,” where he treated Annette and the gang to his precociously adept tap-dancing and traps-playing. Some years later, “Triple-Scale” Gadd brought not only the noize, but also the funk, when he laid down this canonical groove for Little Paulie Simon.
This funky march is pretty much the reference work for a style of drumming that was big in the late 70s and early 80s, “linear drumming,” wherein you never strike two drums at once; the rhythmic line moves from one part of the set to the next. Dave Garibaldi rocked it linear in Tower of Power; Dave Weckl made linear drumming boring and sad.
February 13, 2004
Perspective for Suffering Programmers
An edited transcript of a recent conversation between two programmers whom I know:
Fred: | the javadoc is from a *decompiler*?! |
Ethel: | you're surprised? |
Fred: | it's sad! |
Ethel: | war and poverty are sadder though |
Fred: | yes, true |
Ethel: | so keep it in perspective :-) |
January 16, 2004
Zap.
I cannot stop watching this video of an insane electric arc.
I especially love the tantalising beginning of a "whoop!" from one of the workers as the video abruptly ends. It has the same effect on me as the intentionally tense ending of "Her Majesty" on "Abbey Road."
via MemePool
January 15, 2004
Bush and Mars
Google provides warm fuzzies for nasa:
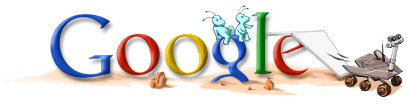
I'm very happy to have witnessed a successful landing on Mars. I'm particularly happy that it was unmanned. I am firmly of the opinion that, if one values science per se, manned missions are a disastrous waste of money.
I am very unhappy, on the other hand, about Bush's ill-conceived push for the moon. There's the matter of his already having bankrupted the nation through vast military-industrial welfare (a.k.a. the "War on Terror"), but there's the more puzzling and frightening matter of motivation. I don't think anyone could seriously entertain the notion that Bush has any personal interest in science. Why does he want the American flag planted on the moon? Is it merely a further gargantuan handout to the military-industrial complex?
December 09, 2003
Da da
My son, who's a bit over 7 months old as of this writing, is a delicious babbler. He's had the bilabial sounds pretty much together for quite some time—"ba ba ba ma ma ma"—but has only recently begun the alveolar plosives—"ta ta ta da da da".
I know that he's babbling; I know that he's not naming anything. But whenever he goes "da da" I just melt.
October 22, 2003
Sucka MC (Win32 Logging Misery)
As part of a distributed system I'm developing for my job at IBM Research, I've written a Win32 application that sits in the background and communicates with a Java program via TCP. I wanted to add some logging to it, just to keep tabs on the various nefarious things it has to do. I figured, "Win32 has to provide some standard API for writing to the event log." I was right. But it's a huge drag. It's very featureful, and provides for localization, and has a great deal of flexibility for customized categories of events, but it's egregiously difficult to hack, and far too heavyweight for "casual" logging.
Continue reading "Sucka MC (Win32 Logging Misery)"October 15, 2003
What children are like.
My wife forwarded me this message from a friend:
A couple of weeks ago I ran into a friend of mine, she was with her little boy, who when I said "let's see how tall you are" he came right up to me and measured his height across my chest, and copped a quick feel. His mom apologized, and went on to tell me this story - they were at the beach with a friend of hers, who is quite well endowed, and is actually a dancer in Texas. Her son, Dakota, snugged up on this large breasted woman's lap, pulled out the top of her bathing suit, looked down, and said "I love you" to her. They are like that from birth.
Now that's a specification.
I was browsing the Javadoc for JSR 166 (Doug Lea's excellent util.concurrent package, slated for Java 1.5), when I encountered this wry caveat in the documentation for the new System.nanoTime() method:
Differences in successive calls that span greater than approximately 292 years (263 nanoseconds) will not accurately compute elapsed time due to numerical overflow.
You have been warned.